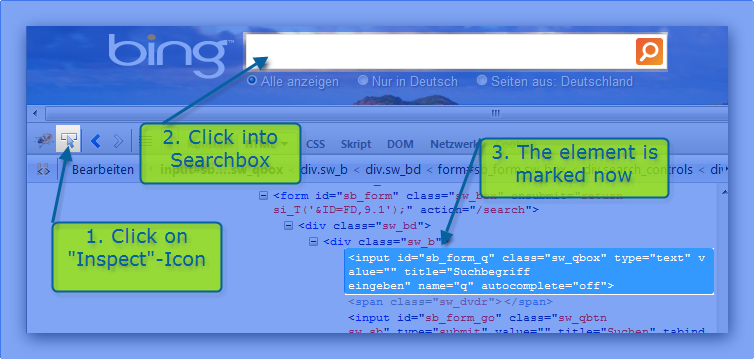
Example how to use Selenium with Visual Studio and Visual Basic.NET
(The code in this example is Visual Basic.net but could of course be easily transferred into C#). So we have set up Selenium (as described here) and followed some general tips (as described here). Now it’s time to write our first unit test in Visual Studio using Visual Basic.net and Selenium. We will do a rather simple test but it covers several aspects of web testing. We will write a test to check whether a link to our current domain dotnet-developer.de is returned as first result from bing when we search for “dotnet-developer.de”. So we have to write a webtest with these steps: Continue reading…
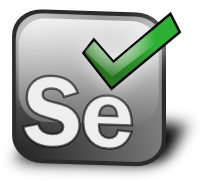
Tips and Tricks for Selenium WebTests
After setting up Selenium for usage in Visual Studio Unit Tests as described in previous posting, now I want to share some tips for writing your unit tests. These tips should help you during the driver initialization and retrieval of web elements.
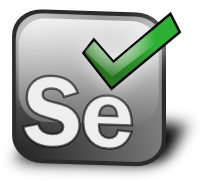
Use Selenium for WebTests with Visual Studio
Unit Tests in Visual Studio are great for testing backend functionality, but it does not help if you want to test websites. Searching around, there are several tools to fill this gap. My favorite is Selenium. It has a Firefox plugin for fast and easy web tests. And it has a WebDriver Version. Using the WebDriver is a powerful option. You could simulate (nearly) everything you do in a browser from your backend code by creating a unit test in C# or Visual Basic.net!
In current post I first want to summarize the steps to install and setup Selenium WebDriver version in Visual Studio.
So these steps are necessary to install Selenium WebDriver: (My favorite browser is Mozilla Firefox. Of course you could use any other browser (like Internet Explorer or Google Chrome) but for easier explanation I’ll just use Firefox here)
- Download the latest .net Selenium drivers from their Google Code Page. Current release is “selenium-dotnet-2.25.1.zip”. Extract the files to your hard disk.
- Open Visual Studio and create a new Test Project. Or add a new Test Project to your existing solution (Add –> New Project –> Testproject)
- Add the following DLLs from the extracted Selenium drivers directory (see step 1):
-
- WebDriver
- WebDriver.Support
-
- Add the following imports to your Test.vb (or Test.cs of course) for easier usage:
- Imports OpenQA.Selenium
- Imports OpenQA.Selenium.Firefox
- Imports OpenQA.Selenium.Support.UI
- Start writing your tests

Log all assemblies used
Sometimes it’s quite helpful to know which assembly is currently used by an application. Microsoft has an example in their MSDN-Article explaining AssemblyName.Version Property. So this article is more a reminder because it’s quite helpful on application initialization to log the assemblies sometimes. But logging should not be done too early, because assemblies are not available as long as they are not used so it would be better to add it at the end of the initialization.
The logger is log4net but could of course easily be changed.
Update 07. January 2014: Yesterday evening several Windows Patches have been deployed on our server. Since then one of our vb.net application did not work anymore. It worked fine with .net 4.0 before but now crashes with the following exception: The invoked member is not supported in a dynamic assembly. The reason is found here: http://bloggingabout.net/blogs/vagif/archive/2010/07/02/net-4-0-and-notsupportedexception-complaining-about-dynamic-assemblies.aspx Strange because the application ran fine so far, but installing patch described in Microsofts KB 2858725 stopped the application from working. So please find below the updated version.
Private Sub LogAllAssemblies(ByVal log As log4net.ILog) Dim MyAssemblies As System.Reflection.Assembly() Dim i As Integer MyAssemblies = System.Threading.Thread.GetDomain().GetAssemblies() log.Info("Current application uses " & MyAssemblies.Length.ToString & " assemblies:") For i = 0 To MyAssemblies.GetUpperBound(0) Try If MyAssemblies(i).GetType().FullName = "System.Reflection.Emit.InternalAssemblyBuilder" Then log.Debug("Reflection is not supported by assembly Nr " & (i + 1).ToString & " so could not log all details for it.") log.Debug((i + 1).ToString & ". " & MyAssemblies(i).GetName().Name & " (Location not supported) - " & _ "From GAC: " & MyAssemblies(i).GlobalAssemblyCache.ToString & _ " - Image Runtime: " & MyAssemblies(i).ImageRuntimeVersion & _ " - Version: " & MyAssemblies(i).GetName().Version.ToString & _ " - Full name: " & MyAssemblies(i).FullName) Else log.Debug((i + 1).ToString & ". " & MyAssemblies(i).GetName().Name & " (" & MyAssemblies(i).Location & ") - " & _ "From GAC: " & MyAssemblies(i).GlobalAssemblyCache.ToString & _ " - Image Runtime: " & MyAssemblies(i).ImageRuntimeVersion & _ " - Version: " & MyAssemblies(i).GetName().Version.ToString & _ " - Full name: " & MyAssemblies(i).FullName) End If Catch ex As Exception log.Debug("Could not log assembly Nr " & (i + 1).ToString & ": " & ex.Message) End Try Next End Sub

Creating Active Directory Contacts using vb.net
I need to create an application syncing 2 Active Directories: For each User in Actice Directory A, a Contact-Document should be created in Active Directory B. .net Framework already provides System.DirectoryServices for this purpose. Doing some tests I got the following System.DirectoryServices.DirectoryServicesCOMException: “The server is unwilling to process the request.” OK, maybe I should wait some minutes until the server is willing to process my request? Or maybe after weekend? That was not an option 😉 Searching around I found several solutions, e.g. properties were not written case-sensitive or typing errors. Continue reading…
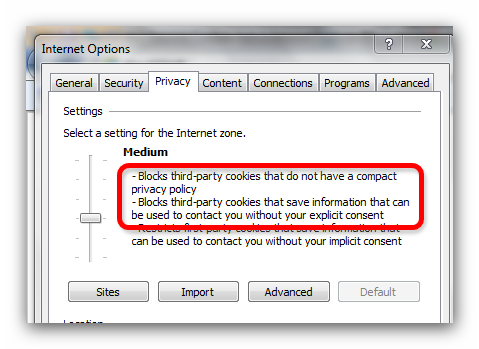
Session Cookies lost in Internet Explorer IE9 ..
or: How to create P3P (privacy policy) Files for IIS.
I have a facebook application which runs within the facebook frame. Everything works fine. I’m using Facebook C# SDK to authenticate the user. Then I store several information, e.g. users name and his facebook id, in session variables. Everything works fine but I betatester complained that he is not able to use the application. Checking his settings shows that he uses IE 9. That should not be the problem, just a side note: All others use Firefox and Chrome…. However, taking a closer look shows that his session cookies are lost! I could store them and immediately retrieve them, but if I want to access them in another part 2 seconds later they are gone. I’ve tried with my own IE 9 and it’s the same result: Session Cookies are lost. Firefox, Chrome and Opera work fine. So what’s the difference with IE? I have another app where each user has to authenticate with Facebook but there the app does not run within the facebook frame but in a seperate window, and there everything is working fine…

Uploading a file with asp.net, jQuery and jTemplate
Playing around with jQuery and jTemplate and I had the following problem: On a page created with jTemplate I could not use asp:FileUpload Control. Additionally it would of course be better to have an Ajax / jQuery control as this would better integrate into a jQuery website. There are several jQuery Fileupload controls, and Ajax-Upload is my favorite, e.g. because it allows multiple fileupload and does not require Flash. Andrew Valums, the creator of the Ajax-Upload, has posted a C#-Example but as it’s just posted in the comments it might be missed easily. Additionally I needed it for Visual Basic so I’ve transfered it and wrote this short blog entry.
First follow the instructions at Ajax-Upload page to setup your page. I use the following JavaScript-Code within my function
var uploader = new qq.FileUploader({ element: document.getElementById('file-uploader'), action: 'MyWebservice.asmx/ProcessFile', debug: false, sizeLimit: 1100000, // max size 1 MB allowedExtensions: ['txt'] });
In the webservice I then use the following code. The code is shortened to the basics, of course you should add a check whether the filename already exists etc. but it should only demonstrate how to save the uploaded file.
Dim Filename As String = "c:\temp\upload.txt" 'get reference to posted file and do what you want with this file If Me.Context.Request.Files.Count = 0 Then 'Firefox does not upload it as file Dim Length As Integer = 4096 Dim BytesRead As Integer = 0 Dim buffer As [Byte]() = New [Byte](Length) {} Try Using fileStream As New IO.FileStream(ImportPath & Filename, IO.FileMode.Create) Do BytesRead = Context.Request.InputStream.Read(buffer, 0, Length) fileStream.Write(buffer, 0, BytesRead) Loop While BytesRead > 0 End Using Catch ex As UnauthorizedAccessException ' log error hinting to set the write permission of ASPNET or the identity accessing the code End Try Else Dim postedfile As HttpPostedFile postedfile = TryCast(Me.Context.Request.Files.[Get](0), HttpPostedFile) postedfile.SaveAs(Filename) End If

Compare XML Values with existing table rows
I have a MS SQL Table containing user data, e.g. Username and ID. This list should be compared with another list which is available as XML Document. Fortunately MS SQL 2005 is able to process XML so I searched around and found a post from Divya Agrawal on MSSQLTips.com. In Divyas tip 2 XML files are compared, so I modified it to compare XML and SQL row.
For this example, my SQL Table ‘Users’ has just 2 rows: “Name” (varchar(100)) and “ID” (int). My XML File looks like this:
<root> <data> <name>Thorsten Test</name> <id>123</id> </data> <data> <name>Peter Pommes</name> <id>124</id> </data> <data> <name>Wiki Pedia</name> <id>125</id> </data> </root>
So I need to check whether ‘root/data/id’ from XML file is already in table column ‘ID’. If not, the name and the ID should be returned. This could be done with this little query:
CREATE PROCEDURE [dbo].[FindNewUsers] @Userlist XML AS BEGIN SET NOCOUNT ON; Select N.value('name[1]', 'varchar(100)') as Username, N.value('id[1]', 'int') as ID from @Userlist.nodes('/root/data') as X(N) where N.value('id[1]', 'int') Not in (select ID from Users) END
That’s it!