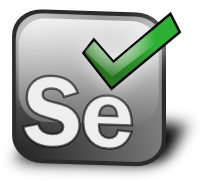
Tips and Tricks for Selenium WebTests
After setting up Selenium for usage in Visual Studio Unit Tests as described in previous posting, now I want to share some tips for writing your unit tests. These tips should help you during the driver initialization and retrieval of web elements.
Initialization
Driver should be initialized only once for all your tests as this makes it easier to switch between different browsers and you don’t have to take care about initialization and termination in each of your tests. Therefore it’s helpful to put the WebDriver Initialization into the Initialization-Sub of your Test class. Additionally you might declare the start URL as a public constant. Here is an example:
<TestClass()> Public Class SeleniumTests Public driver As IWebDriver Const HomePageURL As String = "http://localhost:12345/Default.aspx" <TestInitialize()> Public Sub Initialization() driver = New FirefoxDriver 'driver = New InternetExplorerDriver 'Only necessary for the correct date format, e.g. PublishedDate Thread.CurrentThread.CurrentCulture = New CultureInfo("en") Thread.CurrentThread.CurrentUICulture = New CultureInfo("en") End Sub <TestCleanup()> Public Sub Termination() driver.Quit() End Sub
Get Web Elements
Of course you need to get web elements so for easier access you could use this function.
''' <summary> ''' Waits until the given element is enabled and visible ''' </summary> ''' <param name="webDriver"></param> ''' <param name="definition"></param> ''' <param name="seconds"></param> ''' <returns></returns> ''' <remarks>Needs to wait for .displayed because for e.g. Telerik Treeview all nodes are available but not visible ''' if the parent node is collapsed and therefore the following error would appear: ''' OpenQA.Selenium.ElementNotVisibleException: Element is not currently visible and so may not be interacted with ''' </remarks> Private Function GetWebElement(ByVal webDriver As IWebDriver, ByVal definition As OpenQA.Selenium.By, ByVal seconds As Integer) As IWebElement Dim wait As New WebDriverWait(webDriver, TimeSpan.FromSeconds(seconds)) wait.Until(Function(d) Return d.FindElement(definition).Enabled And d.FindElement(definition).Displayed End Function) GetWebElement = webDriver.FindElement(definition) End Function