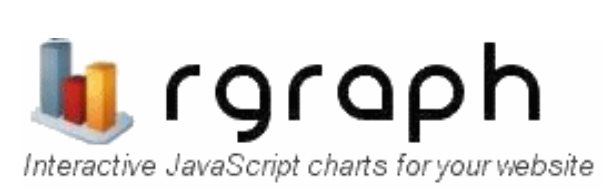
Create HTML5 Charts using ASP.net and RGraph
On an existing web project I wanted to draw some charts: bar charts, pie charts etc. I had a look at Highcharts which looks very good and has some very nice effects (like animation or putting several charts into one) but in an HTML5 book (HTML5 & CSS3 from O’Reilly) I read about RGraph. That’s a free open source javascript library under MIT license so you could use it in every project you want, personal or commercial. It’s using the new canvas tag, see RGraph Homepage for details.
In Richards Support Forum I saw some requests regarding integration into ASP.net, and as there is no summary so far I’ll add it here. In fact it’s very easy to implement it into ASP.net WebForms.
This tutorial shows how to integrate Richards ‘Getting Starting’ example into ASP.net with retrieving the chart data from the backend.
So first follow all the steps described in Richards tutorial and run your ASP.net application, it should display the chart as expected.
Now go to your backend end. You need to provide 2 public properties: ChartData (containing the numeric values) and ChartLabels (containing the chart labels, surprise surprise). Additionally you need 2 private variables containing the data itself (m_chartData and m_chartLabels).
Now you could add your code. I’ve created a new sub ‘SetValues’ which sets the values…. Of course here you could do any operations you like, e.g. retrieve the data from a database or a webservice etc.. Make sure your sub ‘SetValues’ in called on Page_Load. And that’s already all for the Backend!
Here is the complete .aspx.vb code :
Public Class MyWebpage Inherits System.Web.UI.Page Private m_chartData As String Public Property chartData() As String Get Return m_chartData End Get Set(value As String) m_chartData = value End Set End Property Private m_chartLabels As String Public Property chartLabels() As String Get Return m_chartLabels End Get Set(value As String) m_chartLabels = value End Set End Property Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load If Not Page.IsPostBack Then SetValues() End If End Sub Private Sub SetValues() chartData = "[280, 45, 133, 166, 84, 259, 266, 960, 219, 311, 67, 89]" chartLabels = "['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']" End Sub End Class
Of course you also have to make some changes to the .aspx page itself. Locate the script provided by Richard in his 4th step. The data and labels are hardcoded, but now you want to retrieve it from the backend. So tell the script to use the new public properties like this:
$(document).ready(function () { var data = <%=chartData%>; var bar = new RGraph.Bar('myCanvas', data) .set('labels', <%=chartLabels%>) .set('gutter.left', 35) .draw(); });
And you’re done!! The chart should be displayed fine now. Of course this works fine with all other chart types like pie chart, meter charts, line charts etc.. Have fun! And don’t forget to donate to Richard for his excellent piece of code!