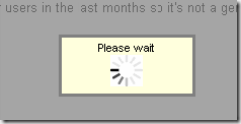
Display ‘Please wait’ popup
This procedure explains how to add a ‘Please wait’ screen to an ASP.net application.
By default at server process (e.g. saving a request) you only see a progress bar in the status bar . If it’s a long running process you might not see any update for a longer time, and users might think that the application stopped, is already finished or did not even start because they did not press the correct button. This might result in double clicks on the Save button or closure of the browser window without completion.
Therefore it is better to display message in the middle of the screen so the user is aware that there is something going on. Current procedure describes how to disable all existing web controls (buttons etc.), coloring all areas in dark gray (fade out) and display an animated circle next to a “Please wait” text.
The procedure has been created based on ASP.net 2005 (2.0) and Microsoft’s Ajax Control Toolkit Release 11119.
CSS
Add the following lines to your CSS file:
/*Modal Popup*/ .modalBackground { background-color:Gray; filter:alpha(opacity=70); opacity:0.7; } .modalPopup { background-color:#ffffdd; border-width:3px; border-style:solid; border-color:Gray; padding:3px; text-align:center; } .hidden {display:none}
JavaScript
Add the following JavaScript Code to your aspx page:
<script type="text/javascript" language="javascript"> function pageLoad(sender, args) { var sm = Sys.WebForms.PageRequestManager.getInstance(); if (!sm.get_isInAsyncPostBack()) { sm.add_beginRequest(onBeginRequest); sm.add_endRequest(onRequestDone); } } function onBeginRequest(sender, args) { var send = args.get_postBackElement().value; if (displayWait(send) == "yes") { $find('PleaseWaitPopup').show(); } } function onRequestDone() { $find('PleaseWaitPopup').hide(); } function displayWait(send) { switch (send) { case "Save": return ("yes"); break; case "Save and Close": return ("yes"); break; default: return ("no"); break; } } </script>
Taking a closer look at the script you see the last function “displayWait”. There you could define on which buttons the ‘Please wait’ screen should be displayed. In current example the button has to have the text “Save” or “Save and Close”. On all other buttons the “Please wait” screen will not be displayed. Of course you should modify this function according to your needs.
Buttons in Ajax UpdatePanel
Add an Update Panel to your aspx page. Place your buttons in the Update-Panel. At least the buttons which should display the ‘Please wait’ screen need to be in the UpdatePanel, but you could of course also add other buttons etc. there.
<asp:UpdatePanel ID="PleaseWaitPanel" runat="server" RenderMode="Inline"> <ContentTemplate> <asp:Button ID="Save" runat="server" Text="Save" OnClick="Save_Click" /> <asp:Button ID="SaveClose" runat="server" Text="Save and Close" OnClick="SaveClose_Click" /> </ContentTemplate> </asp:UpdatePanel>
Aspx Controls
Add a Panel, Button and a ModalPopupExtender to your aspx page. The Panel will be displayed in the middle of the screen. The hidden button is necessary for the ModalPopupExtender as it needs a TargetControl.
<asp:Panel ID="PleaseWaitMessagePanel" runat="server" CssClass="modalPopup" Height="50px" Width="125px"> Please wait<br /> <img src="img/ajax-loader.gif" alt="Please wait" /> </asp:Panel> <asp:Button ID="HiddenButton" runat="server" CssClass="hidden" Text="Hidden Button" ToolTip="Necessary for Modal Popup Extender" /> <ajaxToolkit:ModalPopupExtender ID="PleaseWaitPopup" BehaviorID="PleaseWaitPopup" runat="server" TargetControlID="HiddenButton" PopupControlID="PleaseWaitMessagePanel" BackgroundCssClass="modalBackground"> </ajaxToolkit:ModalPopupExtender>
Image
Add an animated gif to your project. Check Ajaxload.info to create your own if you like, otherwise you might use this one:
Build
That’s it. Now build your application. Or maybe you want to download the example.