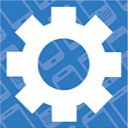
Open App-Settings in Xamarin Forms
Sometimes it’s necessary for the user to grant some access rights, e.g. for camera or photo library. Typically the user is asked on first access, but if he denies he will not be prompted anymore. So you should forward the user to the settings page instead of just saying “Hey, go to your settings page and do the changes”.
For iOS it’s quite easy to do in Xamarin Forms ( Device.OpenUri(new Uri(“app-settings:”));), but there is no such command for Android so you have to use Dependency Service.
On StackOverflow, EvZ has posted the code how to do this. So here are the files you have to create in your Xamarin Forms app, including all the usings etc.
.net standard application
In your .net standard application, add a new interface called “IAppSettingsHelper.cs” with this content (modify Namespace according to your settings):
using System; namespace MyApp.Helpers { public interface IAppSettingsHelper { void OpenAppSettings(); } }
Android App
Create a new class “AppSettingsInterface.cs” in your Android App. Modify “package_name” according to your package, e.g. “com.example.myapp”.
using System; using Android.Content; using MyApp.Droid; using MyApp.Helpers; using Xamarin.Forms; using Application = Android.App.Application; [assembly: Dependency(typeof(AppSettingsInterface))] namespace MyApp.Droid { public class AppSettingsInterface : IAppSettingsHelper { public void OpenAppSettings() { var intent = new Intent(Android.Provider.Settings.ActionApplicationDetailsSettings); intent.AddFlags(ActivityFlags.NewTask); string package_name = "my.android.package.name"; var uri = Android.Net.Uri.FromParts("package", package_name, null); intent.SetData(uri); Application.Context.StartActivity(intent); } } }
iOS App
Finally also create a new class “AppSettingsInterface.cs” in your iOS App. Note that it’s not needed to add your application id here as iOS will automatically open the correct settings.
using System; using MyApp.Helpers; using MyApp.iOS; using Foundation; using UIKit; using Xamarin.Forms; [assembly: Dependency(typeof(AppSettingsInterface))] namespace MyApp.iOS { public class AppSettingsInterface : IAppSettingsHelper { public void OpenAppSettings() { var url = new NSUrl($"app-settings:"); UIApplication.SharedApplication.OpenUrl(url); } } }
From Xamarin Forms, you could now open app settings on Android or iOS just with this command:
DependencyService.Get<IAppSettingsHelper>().OpenAppSettings();
In your Android code you can change:
string package_name = "my.android.package.name";
To:
string package_name = Application.Context.PackageName;
This will give you the default PackageName so that you won’t have to enter it manually.
thanks
I would suggest passing in the bundle-id into the dependency service. The bundle-id can be easily gotten in the cross-platform project using Xamarin.Essentials.AppInfo (https://docs.microsoft.com/en-us/xamarin/essentials/app-information?tabs=ios).
For the iOS implementation, if you add the bundle-id after “app-settings:”, the settings will open to your specific app.
Interface, iOS, and Android implementation code here: https://gist.github.com/jbachelor/d7ff2e61941ae194c5952e6691563dff
Hello, Please how can i open bluetooth setting’s!!?
Hi Benzakour, please have a look at the bluetooth package you are using, e.g. Shiny.
Xamarin.Essentials.AppInfo.ShowSettingsUI();
Thanks