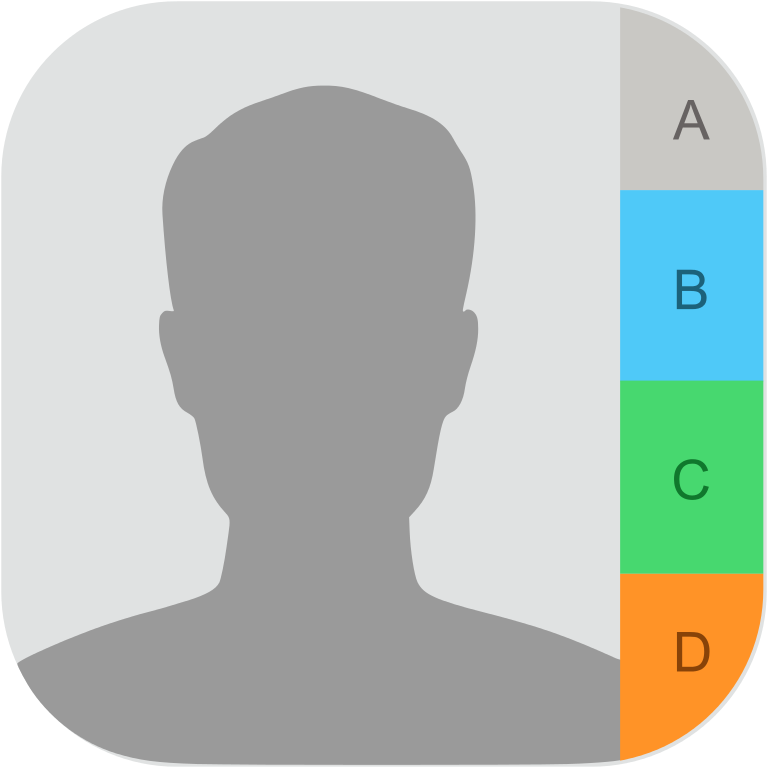
How to read all contacts from local Addressbook using Xamarin Forms
Sometimes it’s a benefit for the user if you could read his contacts and show them in a list so the user could select one or more of them. To read all contacts from a local Addressbook on iOS or Android using Xamarin Forms, James Montemagno has created a Contacts Plugin some time ago.
Be sure to be informed about these information about the Contacts Plugin:
- James does not actively support it anymore (and therefore the github repository shows a notice “This Plugin is not under Development and not supported”)
- The Plugin never left alpha status (latest version is 0.77) .
- It’s not available on nuget anymore.
Nevertheless it does work fine so here are some information how to implement it into your own PCL project.
Install without Nuget
Life is easy with nuget: Select the package, install, done. Now Contacts Plugin is not available on nuget so you have to add the dlls like years ago….
- Download the latest Contacts Plugin from Github.
- Extract the files and open “Contacts.sln” (in src-directory) with Visual Studio.
- Build All in Release mode. (You could not run the project because it does not have any demo project. You only need to build the files).
- In your PCL project, right-click on “References” (not “Packages”) and select “Edit References”.
- Go to tab “.Net Assembly”, click “Browse” and add these 2 files:
- src/Contacts.Plugin/bin/Release/Plugin.Contacts.dll
- src/Contacts.Plugin/bin/Release/Plugin.Contacts.Abstractions.dll
- Do the same within your Android project but use files from “Contacts.Plugin.Android”-Directory.
- Finally do the same within your iOS project and add these 2 files from “Contacts.Plugin.iOSUnified”-Directory.
Get all contacts from local smartphone addressbook
James has posted sample code how to get all contacts from local addressbook. Unfortunately this sample code results in the following error when transfering the result to a list:
System.ArgumentException: Expression of type ‘System.Collections.Generic.IEnumerable`1[Plugin.Contacts.Abstractions.Contact]’ cannot be used for return type ‘System.Linq.IQueryable`1[Plugin.Contacts.Abstractions.Contact]’
Some workarounds are showin in issue 272 of the Contacts Plugin issue tracker. I’m using the idea from flolovebit as the problem does not exist if you first transfer it to the list and afterwards run the query. So the code looks like this:
public static async Task<List<Plugin.Contacts.Abstractions.Contact>>GetLocalContactsFromAddressbook() { List<Plugin.Contacts.Abstractions.Contact> contacts = null; if (await Plugin.Contacts.CrossContacts.Current.RequestPermission()) { try { Plugin.Contacts.CrossContacts.Current.PreferContactAggregation = false;//recommended //run in background await Task.Run(() => { if (Plugin.Contacts.CrossContacts.Current.Contacts == null) return; contacts = Plugin.Contacts.CrossContacts.Current.Contacts.ToList(); contacts = contacts.Where(c => !string.IsNullOrWhiteSpace(c.LastName)).ToList(); }); } catch (Exception ex) { //Do some error handling here } } return contacts; }
Add required settings
Additionally you have to add some further settings to get it running. iOS for example expects a declaration for the enduser why you want to access the contacts. Please have a look at the Contacts Plugin Readme to get all the details.