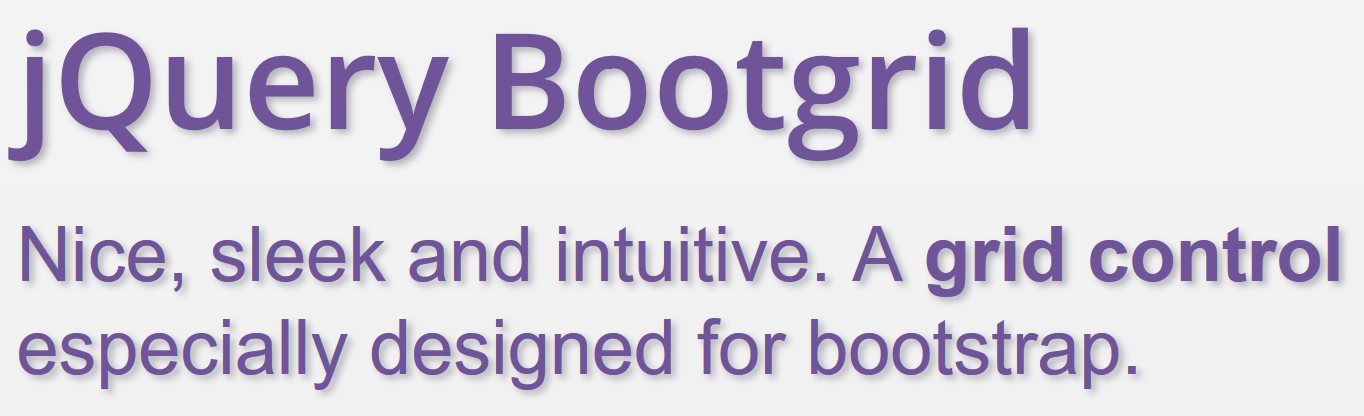
Use jQuery Bootgrid with asp.net Repeater
In last post “Use jQuery Bootgrid with asp.net Gridview” I’ve setup jQuery-Bootgrid to work with an asp.net Gridview control. Now I do the same for asp:Repeater control. Additionally I’ve changed the style of a column and also the output of the datetime column so that it’s now working fine if the datatable contains the value as datetime instead of string.
So the first step is of course the same as in last post:
- Add jQuery, Bootstrap and jquery-Bootgrid Stylesheets and Scripts.
<!-- jQuery, http://jquery.com/ --> <script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript"></script> <script src="//code.jquery.com/jquery-migrate-1.2.1.min.js" type="text/javascript"></script> <!-- Bootstrap, http://getbootstrap.com/ --> <link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.1/css/bootstrap.min.css"> <link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.1/css/bootstrap-theme.min.css"> <script src="//maxcdn.bootstrapcdn.com/bootstrap/3.3.1/js/bootstrap.min.js" type="text/javascript"></script> <!-- Bootgrid, http://jquery-bootgrid.com/ or https://github.com/rstaib/jquery-bootgrid --> <script src="//cdnjs.cloudflare.com/ajax/libs/jquery-bootgrid/1.1.4/jquery.bootgrid.min.js" type="text/javascript"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/jquery-dateFormat/1.0/jquery.dateFormat.min.js" type="text/javascript"></script> <link rel="Stylesheet" href="//cdnjs.cloudflare.com/ajax/libs/jquery-bootgrid/1.1.4/jquery.bootgrid.min.css" />
But already the second step is different: Of course you need to inform Bootgrif about the repeater to be prepared, but if you add ‘data-toggle’ to the repeater you will get the following error message on compiling: ‘Type ‘System.Web.UI.WebControls.Repeater’ does not have a public property named ‘data-toggle’.’ Because when using a repeater you could of course set the attributes (e.g. data-column-id, data-converter, data-formatter etc.) directly within the table structure.
- Inform Bootgrid about the grid to be prepared. 2 Steps are necessary: 1. Add data-toggle=”bootgrid” to your table-definition, and 2. Activate Bootgrid on your gridview. Additionally I personally don’t like case-sensitivity so I turned it off, of course that’s up to you.
<script type="text/javascript" language="javascript"> $(function () { $('#myRepeaterTable').bootgrid({ caseSensitive: false }); }); </script>
- Add data-grid-id for all columns. Same as above, do it directly in the table defintion
<HeaderTemplate> <table class="table table-bordered" id="myRepeaterTable" data-toggle="bootgrid"> <thead> <tr> <th data-column-id="companyname"> Company </th> <th data-column-id="customernumber" data-align="right" data-converter="numeric" data-header-css-class="customerColumn"> CN </th> <th data-column-id="creationdate" data-converter="datetime"> Creation Date </th> <th data-column-id="search" data-formatter="link" data-sortable="false"> Search </th> </tr> </thead> <tbody> </HeaderTemplate>
- Take care about your table structure, especially make sure to use thead and tbody.
Now you should be able to compile your application and it should work fine. One strange thing: The search-link contains several blanks before the company name, therefore I added a trim to remove them. In the previous post you also found information how to use numeric columns, date columns and link columns. This is of course the same regardless whether you use gridview or repeater. The only difference: You could write the data-attributes directly into the HTML and do not need to use any backend databound events or else.
How to sort datetime columns
In previous post I’ve transferred the datetime-column into a string column already in the backend. But sometimes that’s a bit complicated because normally you get the data from an external data source like MS SQL Server, and there your data is hopefully well formatted so that a datetime value is stored in a datetime column.So as you see in ‘GetDatatable’, column ‘CreationDate’ is now datetime instead of string. Therefore in .aspx we need to create a datetime value understable for bootgrid javascript. The Standard Date and Time Format Strings from MSDN offer several parameters which might work fine, e.g. ‘o’ (Round-trip date/time pattern), ‘s’ (Sortable date/time pattern) or ‘u’ (Universal sortable date/time pattern). But none of them worked in all browsers (tested IE, Firefox and Chrome) with all language settings (tested en-US, en-GB and de-DE) and all server language settings (tested en and de), even though Mozilla Documentation about Javascript Date says that “a version of ISO 8601” is supported but nevertheless ‘o’ and ‘s’ failed.. The only formatting which worked finally in all combinations is “R” (RFC1123 pattern) so always take care to format your date with this parameter! I’ve also changed the output of the datetime-converter. You could use default functions like ‘toLocaleDateString’, and you could also combine them with them options but that’s not supported by all browsers, see Mozilla Documentation. Therefore I decided to use jQuery Dateformat library as jQuery is already loaded. Feel free to use any other library likeMoment.js, just depends on your needs.
<th data-column-id="creationdate" data-converter="datetime"> Creation Date </th> <td> <%# Eval("CreationDate", "{0:R}")%> </td> converters: { datetime: { from: function (value) { return new Date(value); }, to: function (value) { return ($.format.date(value, "dd.MM.yy hh:mm")); } } }
How to style columns
So that one is really obvious: In each column you could set ‘data-css-class’ or ‘data-header-css-class’. See my little example in the Customer Number column.
<style type="text/css"> .customerColumn { width: 80px; } </style> <th data-column-id="customernumber" data-header-css-class="customerColumn"> CN </th>
Source Code
Same as in previous post, here is the whole source code:
WebForm1.aspx
<%@ Page Title="" Language="vb" AutoEventWireup="false" MasterPageFile="~/Site1.Master" CodeBehind="WebForm1.aspx.vb" Inherits="WebApplication1.WebForm1" %> <asp:Content ID="Content1" ContentPlaceHolderID="head" runat="server"> <!-- jQuery, http://jquery.com/ --> <script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript"></script> <script src="//code.jquery.com/jquery-migrate-1.2.1.min.js" type="text/javascript"></script> <!-- Bootstrap, http://getbootstrap.com/ --> <link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.1/css/bootstrap.min.css"> <link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.1/css/bootstrap-theme.min.css"> <script src="//maxcdn.bootstrapcdn.com/bootstrap/3.3.1/js/bootstrap.min.js" type="text/javascript"></script> <!-- Bootgrid, http://jquery-bootgrid.com/ or https://github.com/rstaib/jquery-bootgrid --> <script src="//cdnjs.cloudflare.com/ajax/libs/jquery-bootgrid/1.1.4/jquery.bootgrid.min.js" type="text/javascript"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/jquery-dateFormat/1.0/jquery.dateFormat.min.js" type="text/javascript"></script> <link rel="Stylesheet" href="//cdnjs.cloudflare.com/ajax/libs/jquery-bootgrid/1.1.4/jquery.bootgrid.min.css" /> <script type="text/javascript" language="javascript"> $(function () { $('#myRepeaterTable').bootgrid({ caseSensitive: false, formatters: { "link": function (column, row) { return "<a href=\"http://www.bing.com/search?q=" + row.search.trim() + "\">" + column.id + ": " + row.companyname + "</a>"; } }, converters: { datetime: { from: function (value) { return new Date(value); }, to: function (value) { return ($.format.date(value, "dd.MM.yy hh:mm")); } } } }); }); </script> <style type="text/css"> .customerColumn { width: 80px; } </style> </asp:Content> <asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" runat="server"> <asp:Repeater ID="MyRepeater" runat="server"> <HeaderTemplate> <table class="table table-bordered" id="myRepeaterTable" data-toggle="bootgrid"> <thead> <tr> <th data-column-id="companyname"> Company </th> <th data-column-id="customernumber" data-align="right" data-converter="numeric" data-header-css-class="customerColumn"> CN </th> <th data-column-id="creationdate" data-converter="datetime"> Creation Date </th> <th data-column-id="search" data-formatter="link" data-sortable="false"> Search </th> </tr> </thead> <tbody> </HeaderTemplate> <ItemTemplate> <tr> <td> <%# Eval("CompanyName")%> </td> <td> <%# Eval("CustomerNumber")%> </td> <td> <%# Eval("CreationDate", "{0:R}")%> </td> <td> <%# Eval("Search") %> </td> </tr> </ItemTemplate> <FooterTemplate> </tbody> </table> </FooterTemplate> </asp:Repeater> </asp:Content>
WebForm1.aspx.vb
Public Class WebForm1 Inherits System.Web.UI.Page Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load If Not Page.IsPostBack Then With MyRepeater .DataSource = GetDatatable() .DataBind() End With End If End Sub Private Function GetDatatable() As DataTable Dim dt As New DataTable dt.Columns.Add("CompanyName") dt.Columns.Add("CustomerNumber") dt.Columns.Add("CreationDate", GetType(DateTime)) dt.Columns.Add("Search") Dim row As DataRow row = dt.NewRow row("CompanyName") = "Company abc" row("CustomerNumber") = 100 row("CreationDate") = Today.AddMonths(-1) row("Search") = "abc" dt.Rows.Add(row) row = dt.NewRow row("CompanyName") = "Company def" row("CustomerNumber") = 1001 row("CreationDate") = Now.AddDays(-45) row("Search") = "def" dt.Rows.Add(row) row = dt.NewRow row("CompanyName") = "company ghi" row("CustomerNumber") = 70 row("CreationDate") = Now.AddDays(-25) row("Search") = "ghi" dt.Rows.Add(row) row = dt.NewRow row("CompanyName") = "cOmPaNy jkl" row("CustomerNumber") = 345 row("CreationDate") = Now.AddDays(-25).AddHours(3) row("Search") = "jkl" dt.Rows.Add(row) Return dt End Function End Class
Site1.Master
<%@ Master Language="VB" AutoEventWireup="false" CodeBehind="Site1.master.vb" Inherits="WebApplication1.Site1" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> <asp:ContentPlaceHolder ID="head" runat="server"> </asp:ContentPlaceHolder> </head> <body> <form id="form1" runat="server"> <div> <asp:ContentPlaceHolder ID="ContentPlaceHolder1" runat="server"> </asp:ContentPlaceHolder> </div> </form> </body> </html>