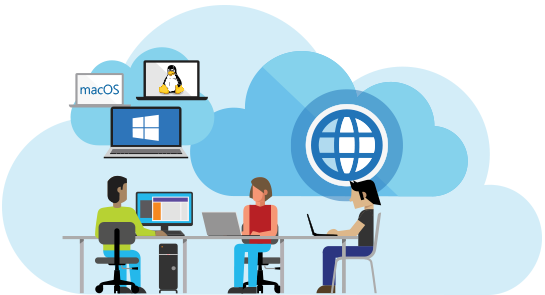
Add new REST Service into existing ASP.net web application
If you want to create a new webservice, you might use the new de-facto standard REST instead of previous solutions from Microsoft like SVC or ASMX. So here are some helpful information if you want to add your first REST Webservice into an existing ASP.net application.
Setup new REST Service in ASP.net
- Create a new folder in your asp.net application
It does not matter how you name the folder, it’s just to separate it from your other files. You could name the folder “Webservices” or “REST” or whatever you want. - Add new item “Web API Controller Class (v2.1)”
Right-click your new folder and select “Add new item”. Choose “Web API Controller Class (v2.1)” which is located in “Web” / “Web API”. Take care about the name of the new class! If you want to have REST urls like “/api/users/”, you need to name your new controller “UsersController” because IIS will automatically take the name of the resource (in current case “users”) and add “Controller” to it. Therefore it’s very important to choose the correct name here. - Update global.asax
While web.config is automatically updated as soon as you add your first Web API Controller Class, you manually need to update your Global.asax. Just add the new route to the “Application_Start” block. Here is an example which allows an optional ID to be supplied so you could call either “/api/users/” or else “/api/users/17” which will be processed in different functions in your UsersController:Web.Routing.RouteTable.Routes.MapHttpRoute(name:="DefaultApi", routeTemplate:="api/{controller}/{id}", defaults:=New With { Key .id = System.Web.Http.RouteParameter.[Optional]})
Code new REST Service in ASP.net
So now the setup is done and it’s time to create your webservice. Here are some hints when writing the code of your new webservice in ASP.net.
- Do not serialize your object in code
Maybe you also use JSON.net, maybe not. However, don’t serialize your return value manually! You don’t need to do something like “JsonConvert.SerializeObject” because asp.net / iis will automatically do this for you. Just return the value you want to return, e.g. a string:Public Function GetExampleString() as String Return "Testvalue" End Function
That’s it. No manual serialization necessary. If you manually serialize your return value, you will get additonal backslashes in your result. Let’s say you replace the above return statement with this one:
Return JsonConvert.SerializeObject(“Testvalue”)
This would serialize your return value twice. Therefore you will see in your receiving code something like “””\””Testvalue\””””” and result in error or at least unexpected values because the string would not be “Test” but “””Test”””. So to avoid these backslashes, don’t serialize manually!
How to get supplied parameters from Request Header
As there are many cases where you need to retrieve values supplied by the calling application from the RequestHeader, here is a function to do that:
Friend Shared Function GetParameterFromHeader(ParameterName As Parameters) As String Dim headerList As NameValueCollection = HttpContext.Current.Request.Headers Dim parameterValue As String If headerList.HasKeys Then If headerList.AllKeys.Contains(ParameterName.ToString) Then parameterValue = headerList.Get(ParameterName.ToString) Else parameterValue = String.Empty End If Else parameterValue = String.Empty End If Return parameterValue End Function
Further interesting resources are
- Microsofts Virtual Academy Course about Web API Design JumpStart
- Using Web API with ASP.NET Web Forms by Mike Wasson
- Routing in ASP.NET Web API also by Mike Wasson