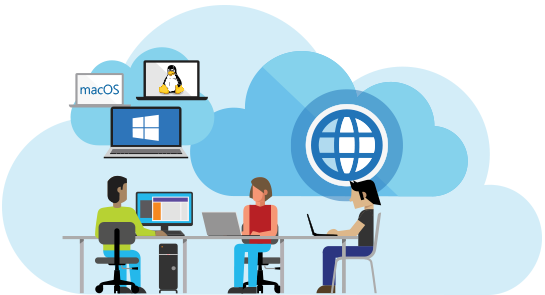
Setup POST REST Webservice with ASP.net and VB.net
Post Add new REST Service into existing ASP.net web application shows how to setup a REST Service to GET data from the Webservice. Typically you also want to write some data back to the server, e.g. when you create a new record you should send a POST request. Here is a description how to do this with visual basic.net.
Probably you already have a CustomersController in your asp.net application in order to retrieve data about your customers. To store new customers, just add a new function called ‘PostCustomer’ to your existing controller. It’s important to name the new function starting with ‘Post’ because the application will handle all POST-Requests for this controller in this function. (You could also name it ‘PostValue’ or ‘PostWhatever’ if you like as long as you start with ‘Post’).
Supply the values
In the customer example you want to supply several information about the new customer, let’s say Firstname, Lastname and CustomerID (In most cases CustomerID will be computed on the server but I just add it here to show you could supply different data types). If you don’t have a class for it already, you could define it now right within your CustomersController, for example:
Public Class CustomersRest Public FirstName As String Public LastName As String Public CustomerID As Guid End Class
So now we create the new function. The data is retrieved from the body, and we will return a response message whether everything worked fine so the calling application knows how to proceed. Within the function we call another backend function ‘db.AddNewCustomer’ which expects 3 parameters: FirstName (String), LastName (String) and CustomerID (GUID). Of course you have to create this function on your own depending on your data structure.
Public Function PostCustomer(<FromBody()> ByVal value As CustomersRest) As HttpResponseMessage If ModelState.IsValid Then db.AddNewCustomer(value.FirstName, value.LastName, value.CustomerID) Return New HttpResponseMessage(HttpStatusCode.OK) Else Return New HttpResponseMessage(HttpStatusCode.BadRequest) End If End Function
So that’s it already.
Call REST POST Webservice
Now that the webservice is created, we could start writing the calling procedure. Here is again a rather simple example. Some information about this example:
- As we don’t know how long the webservice might take to do all the processing, we will do an async call to not stop processing on client side.
- As CustomerID we just supply an empty GUID.
- You need to add Newtonsoft.Json to your import list because we need to serialize the object.
- Make sure to supply correct encoding and type (“application/json”), otherwise you will get error “UnsupportedMediaType”
- Of course you should handle the returned StatusCode to see whether your POST request was processed successfully.
Private Async Sub PostMessageTest_Click(sender As Object, e As EventArgs) Handles PostMessageTest.Click Dim c As New CustomersRest c.FirstName = "Bill" c.LastName = "Gates" c.CustomerID = Guid.Empty Dim RestURL As String = "https://example.com/api/customers/" Dim client As New Http.HttpClient Dim JsonData As String = JsonConvert.SerializeObject(c) Dim RestContent As New Http.StringContent(JsonData, Encoding.UTF8, "application/json") Dim RestResponse As Http.HttpResponseMessage = Await client.PostAsync(RestURL, RestContent) ResultMessage.Text = RestResponse.StatusCode.ToString End Sub
Yes
thanks…………………