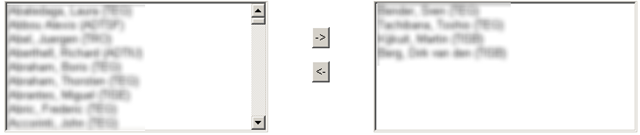
Using JavaScript on listboxes in ASP.net
In my application I needed to have 2 listboxes and users should be able to move entries from the source list to the target list.
Using normal buttons would cause a postback on every click which is ugly of course. Additionally this could be done with JavaScript without problems. The only handicap: As the selection is done in the FrontEnd only, the Backend does not know about it and therefore you could not get the selections made. I’ve found several tips but no complete code so I put together some tips and post it here so you could easily copy it into your application.
The script is based on a blog entry from Colt Kwong: Move Items between 2 Listboxes. But as said above, this script does not give the selected items to the Backend. Searching further I found tips to use a hidden field, and that’s what I’ve done. The script needs to check whether the selected item is already in the hidden list (and so it would remove it) or whether it’s not in the list (and so it will be added).
The JavaScript code:
<script type="text/javascript" language="javascript"> function MoveItem(ctrlSource, ctrlTarget) { var Source = document.getElementById(ctrlSource); var Target = document.getElementById(ctrlTarget); if ((Source != null) && (Target != null)) { while ( Source.options.selectedIndex >= 0 ) { var HiddenList = document.getElementById('<%= SelectedAdditionalInvolved.ClientID %>') //The hidden field var SelectedValue = Source.options[Source.options.selectedIndex].value + ','; // Hidden List is comma seperated var newOption = new Option(); // Create a new instance of ListItem newOption.text = Source.options[Source.options.selectedIndex].text; newOption.value = Source.options[Source.options.selectedIndex].value; Target.options[Target.length] = newOption; //Append the item in Target Source.remove(Source.options.selectedIndex); //Remove the item from Source if (HiddenList.value.indexOf(SelectedValue) == -1) { HiddenList.value += SelectedValue; // Add it to hidden list } else { HiddenList.value = HiddenList.value.replace(SelectedValue,""); // Remove from Hidden List } } } } </script>
In my aspx:
<span class ="selectionListUsernames"> <asp:ListBox ID="AdditionalUsers" Width="100%" Height="100%" runat="server" AutoPostBack="false" /> </span> <span class="selectionListUsernamesButtons"> <br /> <br /> <input onclick="Javascript:MoveItem('<%= AdditionalUsers.ClientID %>', '<%= AdditionalUserSelection.ClientID %>');" type="button" value="->" /> <br /> <br /> <input onclick="Javascript:MoveItem('<%= AdditionalUserSelection.ClientID %>', '<%= AdditionalUsers.ClientID %>');" type="button" value="<-" /> </span> <span class="selectionListUsernames"> <asp:ListBox ID="AdditionalUserSelection" width="100%" Height="100%" runat="server" AutoPostBack="false" /> </span> <asp:HiddenField ID="SelectedAdditionalInvolved" runat="server" />
In my css:
.selectionListUsernames { float:left; width: 300px; height: 150px; } .selectionListUsernamesButtons { float: left; width: 120px; text-align: center; vertical-align:middle; }
In your postback (e.g. when user clicks ‘Save’) you could grab the Hidden field ‘SelectedAdditionalInvolved’. It contains a comma-seperated field containing the selected values, e.g. ‘Value1,Value2,Value3,’. That’s it. If a user selected some values and you saved it make sure to fill the hidden field on next Page_Load.