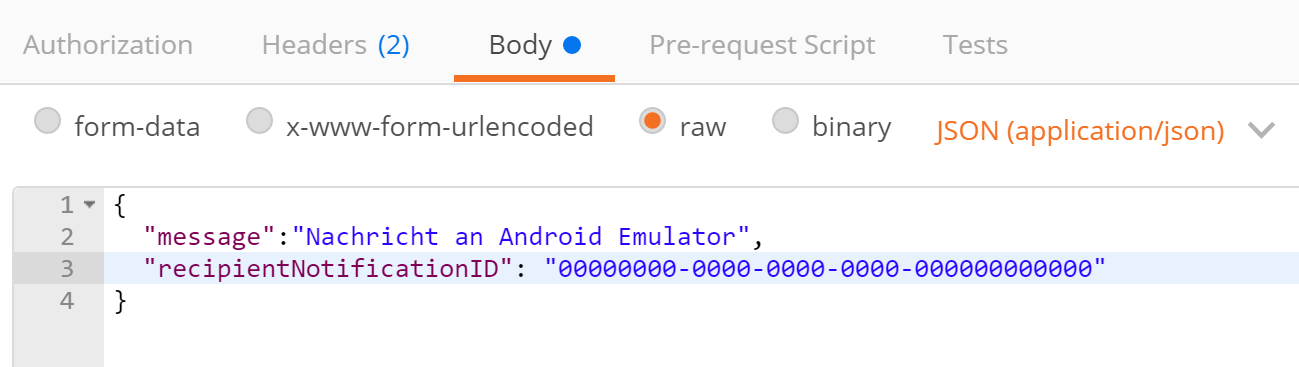
Sending Xamarin Forms Push Notification to single recipient
In previous postings we have setup a Xamarin Forms App to send push notifications via Azure. The next step is now to reduce the number of recipients. Typically we don’t want to send a push notification to all our users. Fortunately only some new lines have to be added to the existing code.
So here I only show the new lines which need to be added to the existing code. If you haven’t seen the previous postings, check them now.
We have to change the Xamarin Forms App and the Azure Mobile App. Xamarin Forms App has to store the individual ID for current user device and needs to send it to the Azure Mobile App together with the message.
Update Xamarin Forms App
We only have to add 2 lines to our Xamarin Forms app. First we have to add a new static Registration ID to the PushClient.cs in the PCL. So just add this definition to the class PushClient right after defining the ApplicationURL constant in line 10:
public static string RegistrationID;
Now we need to set the value. We will do this in the Register-Block in GcmService.cs. The code has already the line where it logs the push.InstallationID to the log in line 64. Right after it we also set the registration ID in our PushClient.cs:
PushClient.RegistrationID = push.InstallationId.ToString();
This is only one example where to store the individual Push Notification ID for this device. It’s up to you to store it e.g. in a database, in local settings etc. Depends on your needs of course.
Update Azure Mobile App
As we need to send the stored registration ID to the Azure Mobile App so it knows where to send the message to, we have to prepare it for receiving this ID. So we add some new lines to the existing post-method in our Azure Mobile App. Currently the Post-Method just expects an object called ‘test’. So we want to bring a bit more structure here. Therefore I created a new class first which could be added anywhere in your code. You might create a new PushNotification.cs if you like. The structure is quite simple.
Public Class PushNotification Public message As String Public recipientNotificationID As String End Class
Now go to the Post-Method of your Controller.cs. The method should now expect an object of our new PushNotification Class.
public async Task<HttpResponseMessage> Post([FromBody] PushNotification message)
MessageParam should of course contain the message we sent to the Controller so replace current line with this one:
templateParams["messageParam"] = message.message;
It’s up to you what you want to do if the recipient ID is not specified. Do you want to accept this message and send it to all of your users? Or do you want to reject the message? Would it make sense in your app to send a push notification to everyone? Probably not, so you might want to reject messages without recipient ID to avoid someone hacking your service and bothering your users with spam. Here is an example if you want to accept both types of messages.
To specify the recipients we use tags. In our case we use “$InstallationID”. It should contain the push notification ID we retrieved in our Xamarin Forms App as shown above.
if (!string.IsNullOrWhiteSpace(message.recipientNotificationID)) { string tags = "$InstallationId:{" + message.recipientNotificationID + "}"; // Send the push notification only to the given recipient result = await hub.SendTemplateNotificationAsync(templateParams, tags); } else { // Send the push notification result = await hub.SendTemplateNotificationAsync(templateParams); }
Now deploy it Azure. As always, this is just a simple example how to get it working. Feel free to modify it so you could support multiple recipients or else.
Test with Postman
Previously we have already used Postman to check whether everything is working fine. So we will now just add the recipient ID and see whether it’s working.
If you enter the ID of your android emulator, the message should be received as expected. And if you change the ID, the message should not reach you of course. So now you are able to send push notifications from your Xamarin Forms App to individual users.